Graphics
ROOT provides powerful graphics capabilities for displaying and interacting with graphical object like plots, histograms, 2D and 3D graphical objects, etc. Here the basic functions and principles are presented, which can be applied to graphs (→ see Graphs) and histograms (→ see Histograms).
The basic whiteboard on which an object is drawn is called in ROOT a canvas
(class TCanvas
). A canvas is an area mapped to a window directly
under the control of the display manager.
A canvas contains one or more independent graphical areas: the pads
(class TPad
). A pad is graphical entity that contains graphical
objects. A pad can contain other pads (unlimited pad hierarchy). A pad is a linked list of
primitives of any type (graphs, histograms, shapes, tracks, etc.).
Adding an element to a pad is done by the Draw()
method of each class.
Painting a pad is done by the automatic call to Paint()
method of each object in the list of primitives.
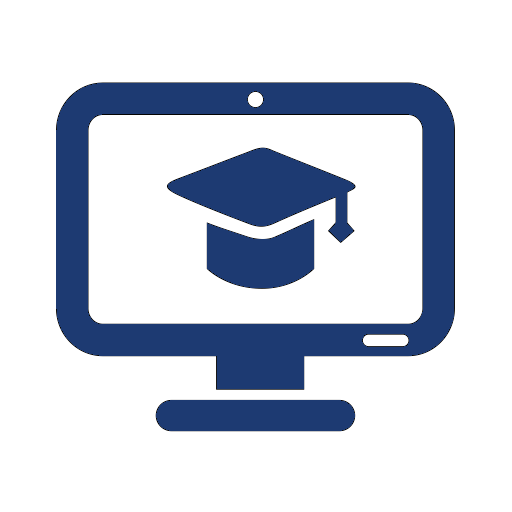
Graphic classesPermalink
ROOT provides numerous graphic classes, of which the following are among the most used:
Working with graphicsPermalink
ROOT offers many possibilities to work with graphics, for example:
- drawing objects
- drawing objects with special characters in its name
- using the context menu for manipulating objects
- using the Graphics Editor for objects
Drawing objectsPermalink
Most of the ROOT classes have a Draw()
method by which they can be “drawn” on a canvas
(TCanvas
class) that contain one or more pads
(TPad
class). When an object is drawn, you can interact with it.
- Use the
Draw()
method to draw an object.
object.Draw()
Example
A one-dimensional sine function shall be drawn.
Use the TF1
class to create an object that is a one-dimensional
function defined between a lower and upper limit.
TF1 f1("func1","sin(x)",0,10)
f1.Draw()
The function is displayed in a canvas.
Figure: Canvas (point to the bottom left light blue square or right-click on the image to interact with the object).
Using the context menu for manipulating objectsPermalink
Right-click on the function to display the context menu.
Figure: Context menu for manipulating objects.
Here you can change many properties of the object like title, name, range, line and fill attributes etc. For example, you can change the range by clicking SetRange
.

Figure: SetRange dialog window.
Select a range, for example 5, 25.

Figure: Range 5, 25 for sin(x).
Using the Graphics Editor for objectsPermalink
You can edit an existing object in a canvas by right-clicking the object or by using the Graphics Editor.
- Click View and then select Editor.

Figure: Editor for setting attributes interactively.
You can draw and edit basic primitives starting from an empty canvas or on top of a picture. There is a toolbar that you can use to draw objects.
- Click View and then select Toolbar.

Figure: Toolbar providing more options.
Graphical objectsPermalink
This section lists some of the graphical objects that ROOT provides. Usually,
one defines these graphical objects with their constructor and draws them with their Draw()
method:
- Lines: Use
TLine
to create a line. - Arrows: Use
TArrow
to create an arrow. - Polylines: Use
TPolyLine
to create a polyline. - Ellipses: Use
TEllipse
to create an ellipse. - Rectangles: Use
TBox
orTWbox
to create a rectangle. - Markers: Use
TMarker
to create a marker. - Curly lines and arcs: Use
TCurlyLine
andTCurlyArc
to create curly lines and arcs for Feynman diagrams. - Text and Latex: Use
TText
to draw simple text.TLatex
for complex text like mathematical formulas. Text can be embedded in a box usingTPaveLabel
,TPaveText
andTPavesText
. - and more …
Colors and color palettesPermalink
Colors are managed by the class TColor
. A color is defined by its
RGB or HLS components. It can be accessed via an
index or
by name for the predefined colors.
Colors can be grouped in palettes. More
than 60 High quality palettes are predefined.
Color can also be transparent.
Graphical objects attributes and stylesPermalink
There are the following classes for changing the attributes of graphical objects:
-
TAttFill : Used for filling an area with color and a style.
-
TAttLine : Used for setting the color, width and style of a line.
-
TAttMarker : Used for setting the color, size and style for a marker.
-
TAttText : Used for setting text attributes like alignment, angle, color, size and font.
Creating and modifying a stylePermalink
When objects are created, their default attributes (taken from TAttFill
, TAttLine
, TAttMarker
, TAttText
)
are taken from the current style. The current style is
an object of the TStyle
class and can be referenced via the global
variable gStyle
(→ see ROOT classes, data types and global variables).
ROOT provides several predefined styles.
Among them: Classic
, Plain
or Modern
(used when ROOT starts).
Setting the current stylePermalink
- Use the
SetStyle()
method, to set the current style.
gROOT->SetStyle("Plain"); // Set the current style to "Plain"
You can get a pointer to an existing style with:
auto style = gROOT->GetStyle("Classic"); // Get a pointer to the "Classic" style.
Note
When an object is created, its attributes are taken from the current style. For example, you may have created an histogram in a previous session and saved it in a ROOT file. Meanwhile, if you have changed the style, the histogram will be drawn with the old attributes. You can force the current style attributes to be set when you read an object from a file by:
gROOT->ForceStyle();
Creating additional stylesPermalink
- Use the
TStyle
constructor to create additional styles.
auto st1 = new TStyle("st1","my style");
st1->Set....
st1->cd(); // This becomes now the current style.
Getting the attributes of the current stylePermalink
You can force objects (in a canvas or pad) to get the attributes of the current style.
canvas->UseCurrentStyle();
AxisPermalink
Axis are automatically built in by various high level objects such as histograms or graphs.
TAxis
manages the axis and is referenced by TH1
and TGraph
.
To make a graphical representation of an histogram axis, TAxis
references
the TGaxis
class.
- Use the
GetXaxis()
,GetYaxis()
orGetZaxis()
methods to get the axis for an histogram or graph.
Example
auto axis = histo->GetXaxis();
Setting the axis titlePermalink
- Use the
SetTitle()
method to set the title of an axis.
Example
axis->SetTitle("My axis title");
If the axis is embedded into a histogram or a graph, you first have to extract the axis object.
Example
histo->GetXaxis()->SetTitle("My axis title")
Setting axis attributesPermalink
The axis graphical attributes are managed via the class TAttAxis
.
Example
auto axis = histo->GetXaxis();
axis->SetLabelColor(kRed); // change the labels'color to red
Setting the number of divisionsPermalink
- Use the
SetNdivisions
method to set the number of divisions for an axis.
Example
auto axis = histo->GetXaxis();
axis->SetNdivisions(510); // Set 10 primary divisions and 5 secondary divisions.
Labels tuningPermalink
Several axis’ attributes can be changed. For instance the size, the distance to the axis, the alignment etc …
SetMaxDigits() set the maximum number of digits permitted for the axis labels above which the notation with 10N is used.
Labels can also be tuning individually thanks to ChangeLabel().
Setting the axis rangePermalink
- Use TAxis::SetRange() or TAxis::SetRangeUser() to zoom the axis.
The SetRange()
method parameters are bin numbers. For example if a histogram plots the
values from 0 to 500 and has 100 bins, SetRange(0,10)
will cover the values 0 to 50.
The SetRangeUser()
method parameters are user coordinates. If the start or end is in the
middle of a bin the resulting range is approximation. It finds the low edge bin for the
start and the high edge bin for the high.
For a general description see the “How to set ranges on axis” FAQ.
Setting time units for axisPermalink
Axis can be labeled with time and date. Such axis are called “Time axis”. A detailed description is given in the TGaxis reference page.
Basically three methods allow to manage such axis:
SetTimeDisplay()
to set an axis as a time axis.SetTimeFormat()
to define the format used for time plotting.SetTimeOffset()
to change the time offset.
Example
{
auto c = new TCanvas("c","Time on axis",0,0,600,400);
// Create and fill some example histogram
auto h = new TH1F("h","Time on X axis",30000,0.,20000.);
for (Int_t i=1;i<30000;i++) {
Float_t noise = gRandom->Gaus(0,120);
h->SetBinContent(i,noise);
}
// Define the X axis as a "Time axis"
TDatime dh(2001,9,23,15,00,00); // Encode a date
auto axis = h->GetXaxis(); // Retrieve the X axis
axis->SetTimeDisplay(1); // X axis will be a "Time axis"
axis->SetTimeFormat("%d %b - %Hh"); // Define the format of the axis labels: day and hour
axis->SetTimeOffset(dh.Convert()); // Set a proper offset
h->Draw();
}
Figure: A simple time axis with day and hour.
Drawing an axis independently of a graph or a histogramPermalink
- Use the
TGaxis
class to draw an axis independently of a graph or a histogram.
This may be useful if you want to draw a supplementary axis for a plot.
LegendsPermalink
A legend is almost always present on a plot. ROOT provides an easy to use tool allowing a direct link between the legend drawn and the legended objects. Therefore, when one of the object attributes is changed, the legend is automatically changed also.
- Use the
TLegend
class to add a legend to graph.
A TLegend
is a panel with several entries (TLegendEntry
class).
The method BuildLegend
automatically build a TLegend
with all the objects present
in a TPad
.
Canvas and padPermalink
A canvas (TCanvas
) is a graphical entity that contains graphical objects that are called
pads (TPad
). A pad is a graphical container that contains other graphical objects like histograms and arrows. It also can contain other pads, called sub-pads. When an object is drawn, it is always in the so-called active pad.
Accessing the active padPermalink
- Use the global variable
gPad
to access the active pad.
For more information on global variables, → see ROOT classes, data types and global variables.
Example
If you want to change the fill color of the active pad to blue, but you do not know the name of the active pad, you can use gPad
.
gPad->SetFillColor(kBlue);
Accessing an object in an active padPermalink
- Use the TPad::GetPrimitive(const char* name) method to access an object in an active pad.
Example
root [0] obj = gPad->GetPrimitive("myobjectname")
(class TObject*)0x1063cba8
A pointer to the object myobjectname
is returned and put into the obj
variable.
The type of the returned pointer is a TObject*
that has a name.
Hiding an object in a padPermalink
You can hide an object in a pad by removing it from the list of objects owned by that pad.
-
Use the TPad::GetListOfPrimitives() method to list is accessible objects of a pad.
-
Use the
Remove()
method to remove the object from the list.
Example
First, a pointer to the object is needed.
Second, a pointer to the list of objects owned by the pad is needed.
Then you can remove the object from the list, i.e. pad.
The object disappears as soon as the pad is updated.
root [1] obj = gPad->GetPrimitive("myobjectname")
root [2] li = gPad->GetListOfPrimitives()
root [3] li->Remove(obj)
Updating a padPermalink
For performance reasons, a pad is not updated with every change. Instead, the pad has a “bit-modified” that triggers a redraw.
The “bit-modified” is automatically set by:
-
touching the pad with the mouse, for example by resizing it with the mouse,
-
finishing the execution of a script,
-
adding or modifying primitives, for example the name and title of an object.
You can set the “bit-modified” by using the Modified()
method.
Example
// The pad has changed.
root [0] pad1->Modified()
// Recursively updating all modified pads:
root [1] c1->Update()
A subsequent call to TCanvas::Update() scans the list of sub-pads and repaints the pads.
Dividing a pad into sub-padsPermalink
To draw multiple objects on a
canvas (TCanvas
), you can it into sub-pads (TPad
).
There are two ways to divide a pad into sub-pads:
Creating a single sub-pad
To build sub-pads in a pad, you must indicate the size and the position of the sub-pads.
Example
A sub-pad is to be built into the active pad (pointed by gPad
). First, the sub-pad is
build using the TPad
constructor.
root [0] auto spad1 = new TPad("spad1","The first subpad",.1,.1,.5,.5)
The NDC (normalized coordinate system) coordinates are specified for the lower left point (0.1, 0.1)
and for the upper right point (0.5, 0.5)
.
Then the sub-pad is drawn.
root [0] spad1->Draw()
For building more sub-pads, repeat this procedure as many times as necessary.
Dividing a pad into sub-pads
- Use the TPad::Divide() method to divide a pad into sub-pads.
Coordinate systems of a padPermalink
For a TPad
the following coordinate systems are available:
You can convert from one system of coordinates to another.
User coordinate system
Most methods of TPad
use the user coordinate system, and all graphic primitives have their parameters defined in terms of user coordinates. By default, when an empty pad is drawn, the
user coordinates are set to a range from 0 to 1 starting at the lower left corner.
- Use the TPad::range(float x1,float y1,float x2,float y2) method to set the user coordinate system.
The argumentsx1
andx2
define the new range in the x direction, andy1
andy2
define the new range in the y direction.
Example
Both coordinates go from -100 to 100, with the center of the pad at (0,0).
root [0] TCanvas MyCanvas ("MyCanvas")
root [1] gPad->Range(-100,-100,100,100)
Normalized coordinate system (NDC)
Normalized coordinates are independent of the window size and of the user system. The coordinates range from 0 to 1 and (0, 0) correspond to the bottom-left corner of the pad.
Pixel coordinate system
The pixel coordinate system is used by functions such as DistanceToPrimitive()
and ExecuteEvent()
. Its primary use is for cursor position, which is always given in pixel coordinates. If (px
,py
) is the
cursor position, px=0
and py=0
corresponds to the top-left corner of the pad, which is the standard convention in windowing systems.
Converting between coordinate systems
TPad
provides some methods to convert from one system of coordinates to another.
In the following table, a point is defined by:
(px,py)
in pixel coordinates,(ux,uy)
in user coordinates,(ndcx,ndcy)
in normalized coordinates,(apx, apy)
in absolute pixel coordinates.
Conversion | Methods (from TPad) | Returns |
---|---|---|
NDC to pixel | UtoPixel(ndcx) | Int_t |
VtoPixel(ndcy) | Int_t | |
Pixel to user | PixeltoX(px) | Double_t |
PixeltoY(py) | Double_t | |
PixeltoXY(px,py,&ux,&uy) | Double_t ux,uy | |
User to pixel | XtoPixel(ux) | Int_t |
YtoPixel(uy) | Int_t | |
XYtoPixel(ux,uy,&px,&py) | Int_t px,py | |
User to absolute pixel | XtoAbsPixel(ux) | Int_t |
YtoAbsPixel(uy) | Int_t | |
XYtoAbsPixel(ux,uy,&apx,&apy) | Int_t apx,apy | |
Absolute pixel to user | AbsPixeltoX(apx) | Double_t |
AbsPixeltoY(apy) | Double_t | |
AbsPixeltoXY(apx,apy,&ux,&uy) | Double_t ux,uy |
Note
All the pixel conversion functions along the Y axis consider that
py=0
is at the top of the pad exceptPixeltoY()
, which assumes that the positionpy=0
is at the bottom of the pad. To makePixeltoY()
converting the same way as the other conversion functions, it should be used the following way (p
is a pointer to aTPad
):
p->PixeltoY(py - p->GetWh());
Setting the Log ScalePermalink
Setting the scale to logarithmic or linear is a pad’s attribute because you may want to draw the same histogram in linear scale in one pad and in log scale in another pad. Setting log scale does not propagate to sub-pads.
TPad defines log scale
for the three directions x
, y
and z
.
Example
root [0] gPad->SetLogx(1); // Set the x axis to log in the current pad
root [1] gPad->SetLogy(0); // Set the y axis to linear in the current pad
Copying a canvasPermalink
- Use the TCanvas::DrawClonePad method to make a copy of the canvas.
You can also use the TObject:DrawClone() method, to draw a clone of this object in the current selected pad.
Printing a canvasPermalink
Once a canvas is created and shows plots ready to be included in a publication as a .png
or a .pdf
image, the Print()
method can be used. All the standard output formats are provided.
Example
auto c = new TCanvas(); // Create a canvas
h->Draw(); // Draw an histogram in the canvas
c->Print("c1.pdf"); // Save the canvas in a .pdf file
Drawing objects with special characters in its namePermalink
In general, avoid using objects that containing special character like \
, /
, #
etc. in the objects names. Also object names starting with a number might be not accessible from the ROOT command line.
/
is the separator for the directory level in a ROOT file therefore an object having a /
in its name cannot be accessed from the command line.
Nevertheless, some objects may be named in this way and saved in a ROOT file. The following macro shows how to access such an object in a ROOT file.
#include "Riostream.h"
#include "TFile.h"
#include "TList.h"
#include "TKey.h"
void draw_object(const char *file_name = "myfile.root", const char *obj_name = "name")
{
// Open the ROOT file.
TFile *file = TFile::Open(file_name);
if (!file || file->IsZombie()) {
std::cout << "Cannot open " << file_name << "! Aborting..." << std::endl;
return;
}
// Get the list of keys.
TList *list = (TList *)file->GetListOfKeys();
if (!list) {
std::cout << "Cannot get the list of TKeys! Aborting..." << std::endl;
return;
}
// Try to find the proper key by its object name.
TKey *key = (TKey *)list->FindObject(obj_name);
if (!key) {
std::cout << "Cannot find a TKey named" << obj_name << "! Aborting..." << std::endl;
return;
}
// Read the object itself.
TObject *obj = ((TKey *)key)->ReadObj();
if (!obj) {
std::cout << "Cannot read the object named " << obj_name << "! Aborting..." << std::endl;
return;
}
// Draw the object.
obj->Draw();
}
3D graphicsPermalink
3D graphics tools for “Event Display”, “Basic 3D” and OPen GL rendering are provided.
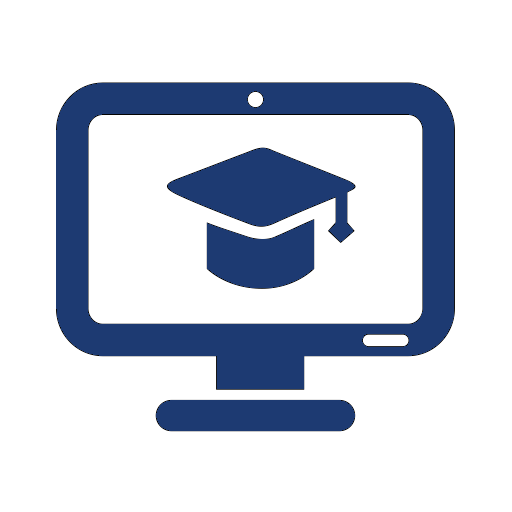
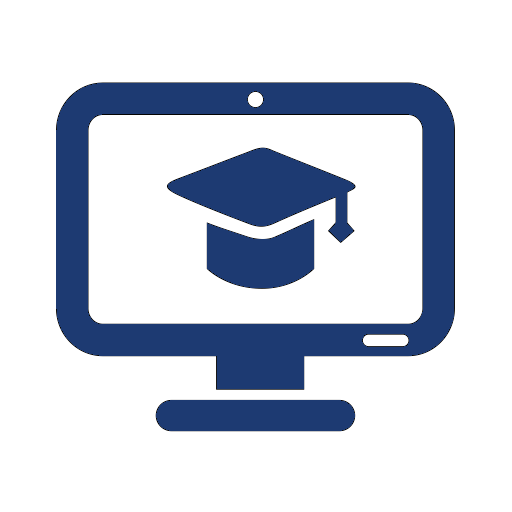